What are fuzzy pixels? Semi-transparent lines that appear on the edges of your Sprites that make them appear fuzzy or blurry. This is the result of positioning a custom drawn Sprite (using the graphics property) on non-whole number pixels (for example myObject.x = 1.5).
Your probably wondering why anyone would set a Sprite to an decimal number of pixels? Well, most of the time this occurs when the positioning is based off of a mathematical equation. For example: myObject.x = this.width / 2. This would produce 50.5 if the width of the container is 101. One of the biggest problems with this is that the fuzzy pixel problem has a waterfall effect. Everything contained within that sprite placed at a decimal number position will have semi-transparent edges. Another reason would be designers that set positioning of items to half pixels in Photoshop and attempting to reproduce the design pixel perfect in Flex.
Here is a zoomed in picture of what fuzzy pixels look like. The first and second squares are drawn using the same drawing function. One is placed on an even pixel and the other at a decimal number.
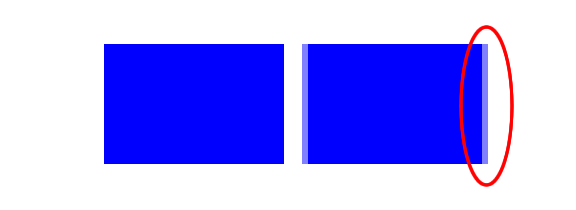
Fuzzy Pixels (Semi-Transparent Lines)
Code that produces fuzzy pixels:
private var fuzzySquare:Sprite; private var square:Sprite; private var squareContainer:Sprite; public function draw():void { squareContainer = new Sprite(); squareContainer.x = 1; squareContainer.y = 1; square = new Sprite(); square.x = 0; square.y = 0; square.graphics.clear(); square.graphics.beginFill(0x0000FF); square.graphics.drawRect(0,0,30,20); square.graphics.endFill(); squareContainer.addChild(square); fuzzySquare = new Sprite(); fuzzySquare.x = square.x + square.width + 3.5; fuzzySquare.y = 0; fuzzySquare.graphics.clear(); fuzzySquare.graphics.beginFill(0x0000FF); fuzzySquare.graphics.drawRect(0,0,30,20); fuzzySquare.graphics.endFill(); squareContainer.addChild(fuzzySquare); container.addChild(squareContainer); }
Solutions:
Use Math.floor or Math.ceil on all mathematical calculations relating to the positioning of Sprites drawn with the graphics function.
or
Rather than draw out the Sprite using graphics you could convert your sprites to images, embed them, and position them on the stage. This is the best approach when tweening is necessary requiring the object to look crisp at all times.
Note: I’ve seen this occur on odd number pixels as well but that is most likely the result of a greater positioning problem. This generally only occurs in highly nested display objects.
use int(x), int(y) – more faster